
Overview
In this article, we will create a sample webpage whose background color is randomly changed when a button gets clicked or when the page is refreshed. We will get the job done by only using JavaScript, HTML, and CSS.
Here’s the demo:
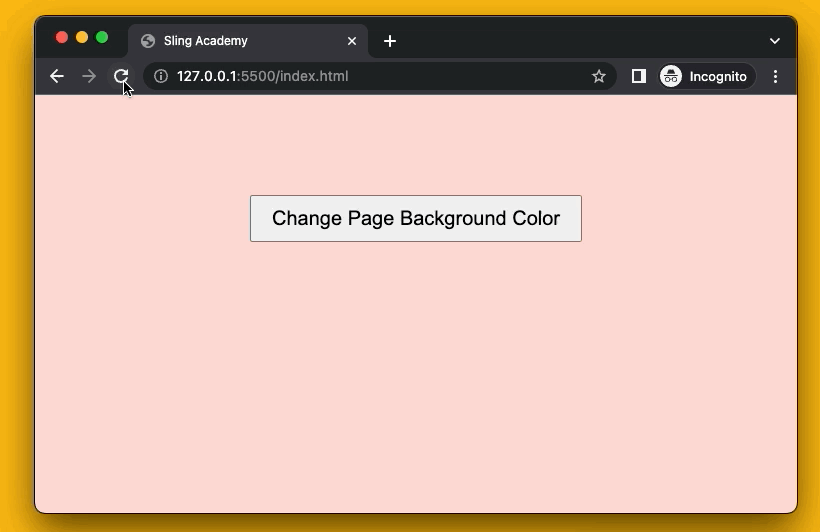
Complete Code
The full source code that produces the example above:
<html>
<head>
<title>Sling Academy</title>
<style>
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
#button {
display: block;
margin: 100px auto;
padding: 10px 20px;
font-size: 20px;
cursor: pointer;
}
</style>
</head>
<body>
<button id="button">Change Page Background Color</button>
<script>
const background = document.getElementsByTagName("body")[0];
const button = document.getElementById("button");
// Function to create random numbers
const getRandomNumber = (maxNum) => {
return Math.floor(Math.random() * maxNum);
};
// Function to create random RGBA colors
const getRandomColor = () => {
const r = getRandomNumber(256);
const g = getRandomNumber(256);
const b = getRandomNumber(256);
const a = Math.random().toFixed(1);
return `rgba(${r}, ${g}, ${b}, ${a})`;
};
// Function to set background color
const setBackgroundColor = () => {
const randomColor = getRandomColor();
background.style.backgroundColor = randomColor;
};
// change background color on button click
button.addEventListener("click", setBackgroundColor);
// change background color on page load/refresh
window.onload = setBackgroundColor;
</script>
</body>
</html>
Save the code in a file name index.html, then open it with your favorite web browser. If you want to find more methods and detailed instructions to create a random color, check this article: How to Generate Random Colors in JavaScript (4 Approaches).
Hope this helps. Happy coding & have a nice day. If you have any questions related to the article, just leave a comment!