This concise article shows you how to get the current date and time in Javascript in yyyy/MM/dd HH:mm:ss format. We’ll write code from scratch without using any third-party libraries.
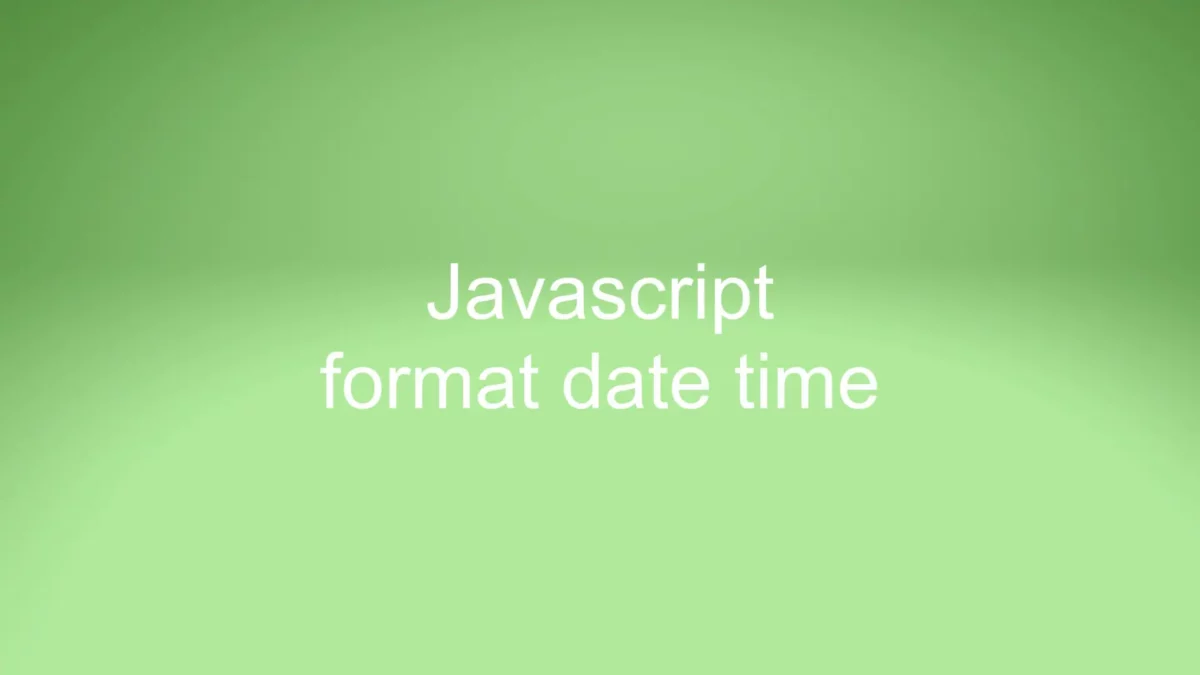
In the example below, we will create a new Date object and then use the getFullYear(), getMonth(), getDate(), getHours(), getMinutes(), and getSeconds() methods to get the current year, month, date, hour, minute, and second, respectively. To make sure the month, date, hour, minute, and second always are in the two-character format, we will use the string method slice().
The code:
const dateObj = new Date();
let year = dateObj.getFullYear();
let month = dateObj.getMonth();
month = ('0' + (month + 1)).slice(-2);
// To make sure the month always has 2-character-format. For example, 1 => 01, 2 => 02
let date = dateObj.getDate();
date = ('0' + date).slice(-2);
// To make sure the date always has 2-character-format
let hour = dateObj.getHours();
hour = ('0' + hour).slice(-2);
// To make sure the hour always has 2-character-format
let minute = dateObj.getMinutes();
minute = ('0' + minute).slice(-2);
// To make sure the minute always has 2-character-format
let second = dateObj.getSeconds();
second = ('0' + second).slice(-2);
// To make sure the second always has 2-character-format
const time = `${year}/${month}/${date} ${hour}:${minute}:${second}`;
console.log(time);
When running the code, you will receive a result like so:
2023/02/24 16:36:13
Note that the method getMonth() returns a value from a range of values from 0 to 11. That’s why we write this line:
month = ('0' + (month + 1)).slice(-2);
You can find more details in Pavel Eliseev’s comment.
I have made every effort to ensure that every piece of code in this article works properly, but I may have made some mistakes or omissions. If so, please send me an email: at [email protected] or leave a comment to report errors.