This practical, example-based article will walk you through a couple of different ways to turn a Map into an object in modern JavaScript (ES6 and newer). I assume you already have some knowledge about the programming language, so I won’t waste your time by explaining what JavaScript is or talking about its history. Let’s get straight to the main points.
Using the Object.fromEntries() method
The Object.fromEntries()
method takes an iterable, such as a Map, and returns an object containing the key-value pairs of the iterable.
Example:
// Create a Map
let myMap = new Map();
myMap.set('website', 'Sling Academy');
myMap.set('platform', 'web');
myMap.set('language', 'English');
// Convert the Map to an object
let myObj = Object.fromEntries(myMap);
console.log(myObj);
Output (in Chrome DevTools console):
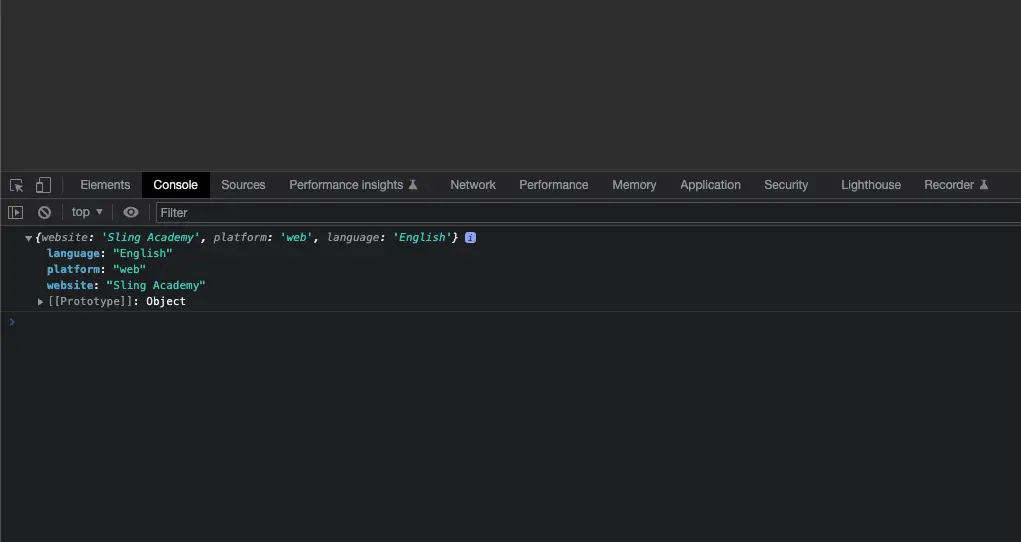
Using the Map.forEach() method
The Map.prototype.forEach()
method executes a callback function for each element in the Map it is called on. In the callback, you can assign each key-value pair to a new object.
Example:
// create a Map
let map = new Map([
["key1", "value1"],
["key2", "value2"],
["key3", "slingacademy.com"]
])
// create an empty object
let obj = {}
// turn the map into an object
map.forEach((value, key) => {
obj[key] = value
})
// print the object
console.log(obj)
Output:
{ key1: 'value1', key2: 'value2', key3: 'slingacademy.com' }
Using a for…of loop
You can use a for...of
loop to the task done by following the following steps:
- Create an empty object that will store the key-value pairs from the input Map.
- Use a
for…of
loop to iterate over the entries of the Map object. Each entry is an array of length 2, containing a key and a value. - In each iteration, use array destructuring to assign the key and value to variables.
- Use bracket notation to assign the key-value pair to the object.
A code example is easier to understand than boring words:
// create a Map
let myMap = new Map([
['key1', 'value1'],
['key2', 'value2'],
['key3', 'slingacademy.com'],
]);
// Create an empty object
let myObj = {};
for (let entry of myMap) {
// Use array destructuring to assign the key and value to variables
let [key, value] = entry;
// Use bracket notation to assign the key-value pair to the object
myObj[key] = value;
}
console.log(myObj);
Output:
{ key1: 'value1', key2: 'value2', key3: 'slingacademy.com' }
Using the old JSON trick
This approach is neither elegant nor efficient. It’s far more lengthy than necessary. I put it here just for reference purposes.
Example:
// create a Map
let myMap = new Map([
['key1', 'value1'],
['key2', 'value2'],
['key3', 'value3'],
]);
// convert Map to JSON
let myStr = JSON.stringify([...myMap]);
// Convert the JSON string to an array of key-value pairs
let myArr = JSON.parse(myStr);
// Use the reduce() method to create an object from the key-value pairs
let myObj = myArr.reduce((acc, [key, value]) => {
// Assign each key-value pair to the accumulator object
acc[key] = value;
// Return the accumulator object
return acc;
}, {}); // The initial value is an empty object
// Log the object
console.log(myObj);
Output:
{ key1: 'value1', key2: 'value2', key3: 'value3' }