SASS (Syntactically Awesome Style sheets) can make your life much easier when writing CSS code with variables, nesting, mixins, and other amazing features. This succinct article shows you how to use SASS in Next.js.
The Steps
1. Install the sass package:
npm i sass -D
2. Add new files with the .scss extension to the styles folder (or rename existing .css files to .scss), like this:
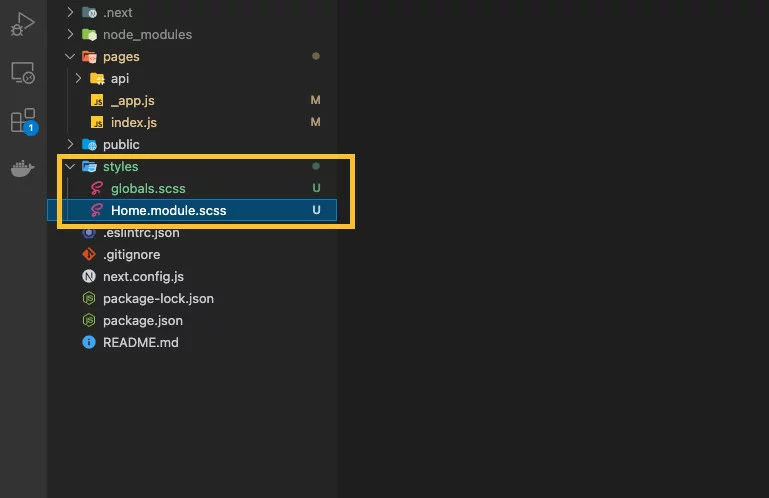
3. Last but not least, update the places where you previously imported css files to scss:
// pages/_app.js
import "../styles/globals.scss";
function MyApp({ Component, pageProps }) {
return <Component {...pageProps} />;
}
export default MyApp;
Example
In this example, we’ll use the nesting feature provided by SASS with BEM naming for classes.
Note: BEM stands for Block, Element, and Modifier. It’s a naming convention whose goal is to facilitate modularity and make styles and classes a lot easier to maintain.
Screenshot:
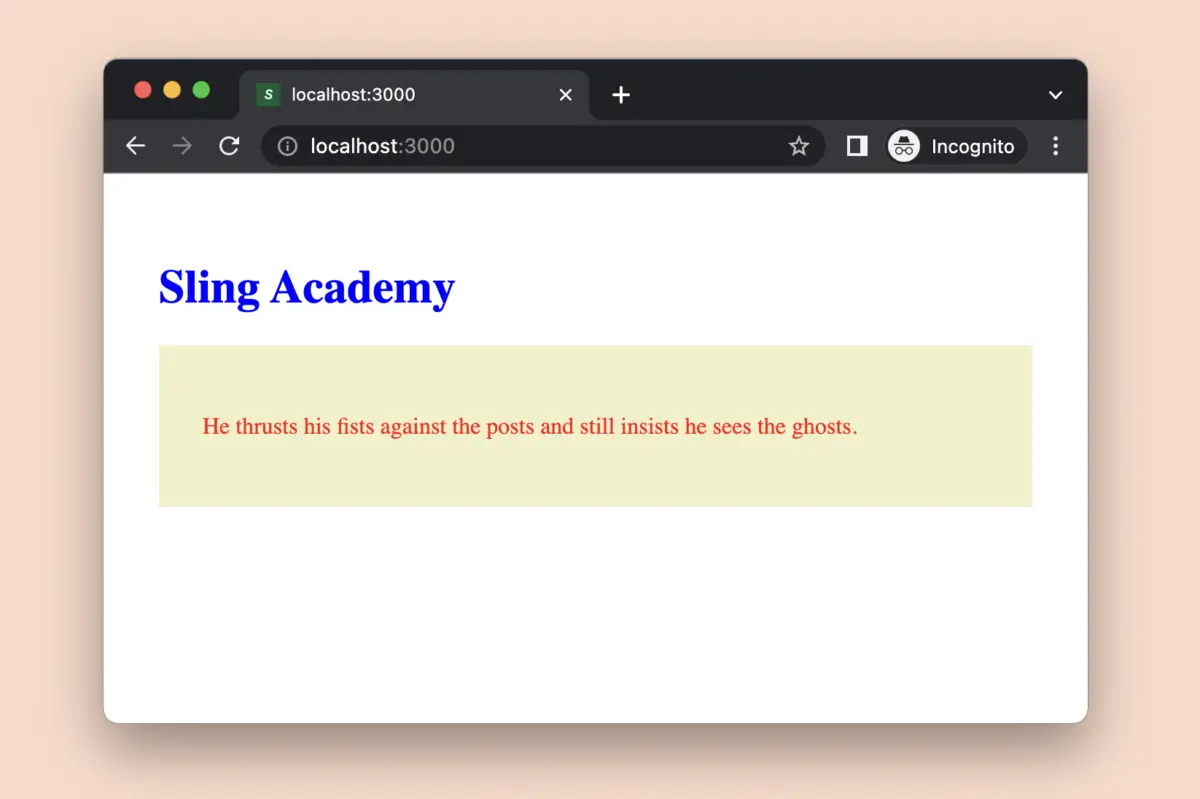
Here’s the code for pages/index.js:
// pages/index.js
import styles from "../styles/Home.module.scss";
export default function Home() {
return (
<div className={styles.home}>
<div className={styles.home__header}>
<h1>KindaCode.com</h1>
</div>
<div className={styles.home__main}>
<p>
He thrusts his fists against the posts and still insists he sees the
ghosts.
</p>
</div>
</div>
);
}
And here is the code in styles/Home.module.scss:
.home {
padding: 30px;
&__header {
h1 {
color: blue;
}
}
&__main {
padding: 30px;
background: rgb(240, 240, 200);
p {
color: red;
}
}
}
That’s it. Leave a comment if you have any questions related to this topic. Happy coding!