In this article, Sling Academy will show you 2 distinct ways to get the URL path of the current route in Next.js 13 or a later version of the framework. The first approach uses the usePathname() hook, and the second approach use __filename (this works for server components). We’ll work with the app directory (which is quite new). No third-party library is required.
Using the usePathname() hook
This technique is simple and quick, but it only works in client components since hooks are not available on the server side. Therefore, you have to add the use client
directive at the very top of your page.
Example:
// app/sling-academy/page.tsx
'use client';
import { usePathname } from 'next/navigation';
export default function SlingAcademy() {
const currentPath = usePathname();
return (
<div style={{ padding: 30 }}>
<h3 style={{ fontSize: 20 }}>
The current path is: <b>{currentPath}</b>
</h3>
</div>
);
}
Screenshot:
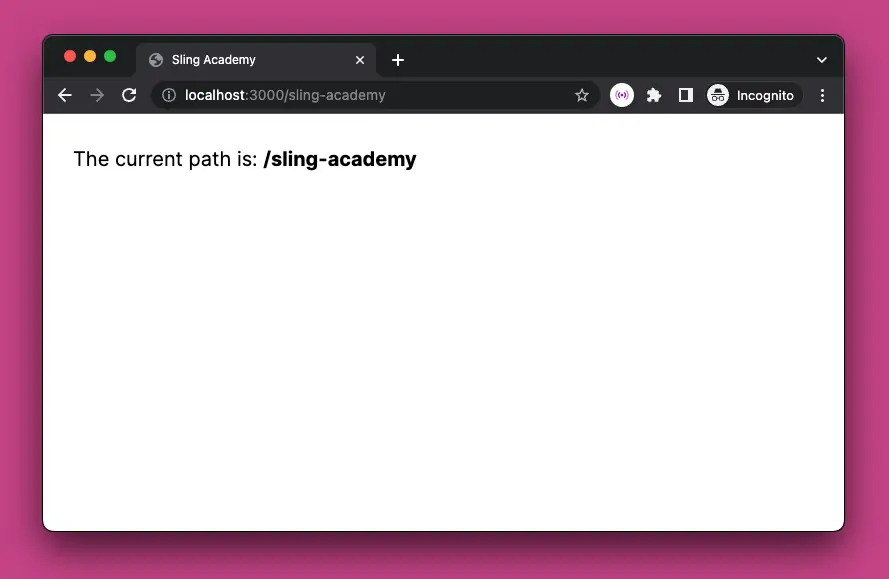
This solution is elegant and concise, but don’t use it in server components, or you will end up with errors and, perhaps, even a headache.
Using the __filename trick (for server components)
This approach is not beautiful as the preceding one, but it works in server components and doesn’t require any third-party libraries.
__filename
is a global variable in Node.js that returns the absolute path of the current file, including the file name and extension. It is ready to use in Next.js’s server components. This variable gives us a long string that looks as follows:
/Users/goodman/Desktop/Dev/next/example-ts/.next/server/app/sling-academy/page.js
What we have to do is to remove unwanted parts from that string to get the current route’s pathname:
.next/server/app
and everything before it.- Everything after the last slash
/
(page.js
,layout.js
, etc).
The built-in split()
and substring()
methods of JavaScript can help us.
Example:
// app/sling-academy/page.tsx
export default function SlingAcademy() {
// get the current file path on the server
const currentFile = __filename
// get the string after ".next/server/app"
let result = currentFile.split(".next/server/app")[1];
// remove everything after the last "/"
result = result.substring(0, result.lastIndexOf("/"));
// see the final result
console.log("result", result);
return (
<div style={{ padding: 30 }}>
<h1 style={{fontSize: 30, color: 'blue'}}>
The current route pathname is: {result}
</h1>
</div>
);
}
Output:
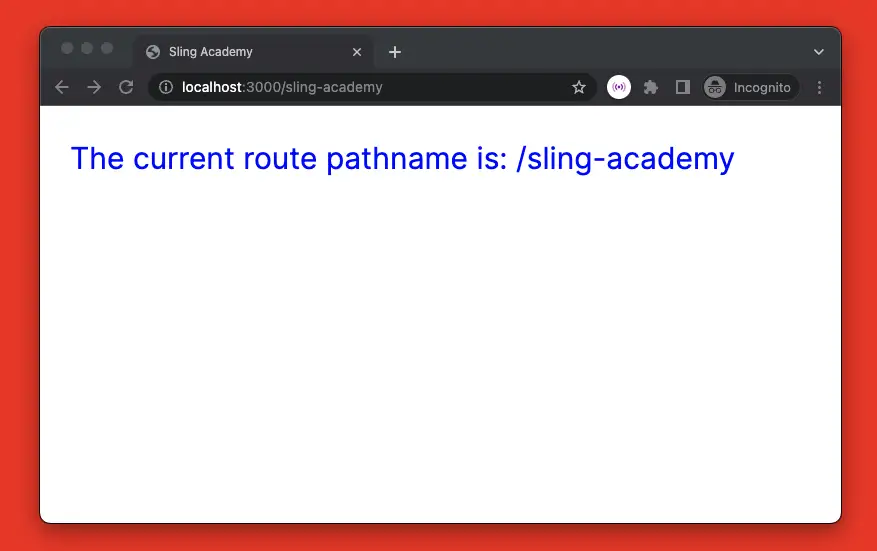
At the time of writing, this is the best and shortest approach I can imagine for reading the current route’s pathname in a sever component in Next.js. Next.js hasn’t provided a built-in solution for this task yet, and in my option, maybe it will never do that.