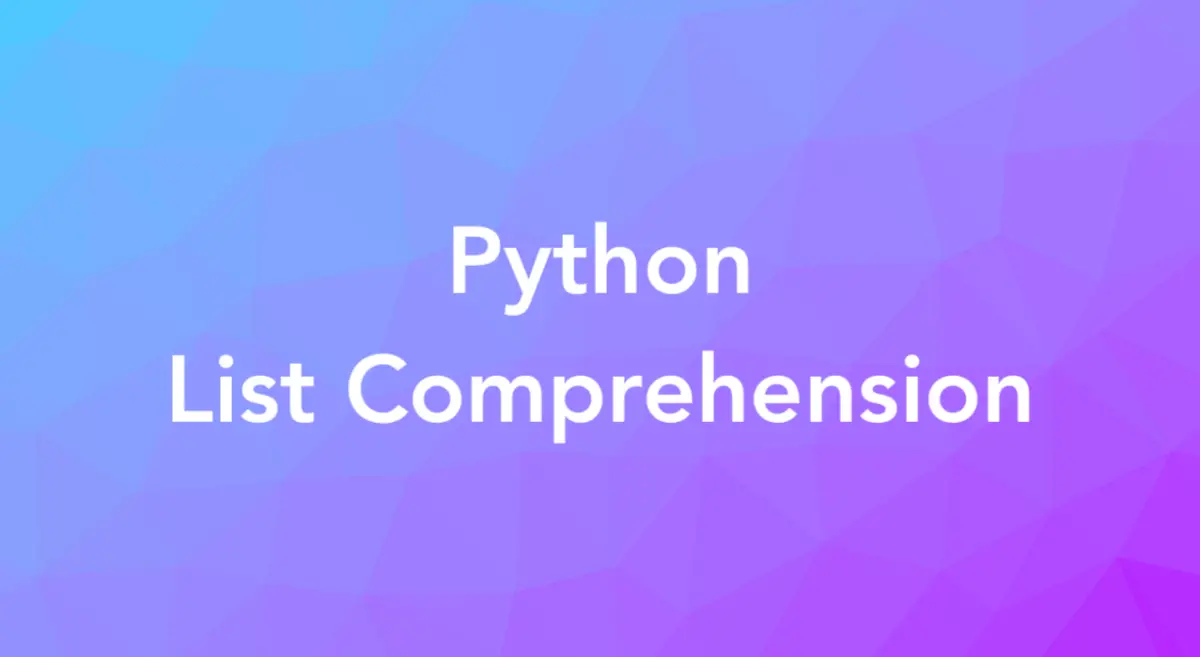
Overview
Python brings to the table an awesome feature that not many popular programming languages have (including JavaScript): list comprehension. It allows you to create new lists by applying transformations or filters to existing iterables in a single line of code.
Syntax:
new_list = [expression for item in iterable if condition]
Where:
new_list
: The new list will be created using list comprehension.expression
: The expression or transformation to be applied to each item in the iterable.item
: It represents each individual item in the iterable that is being processed.iterable
: A sequence or collection of elements (e.g., list, tuple, string) that is being iterated over.if condition
(optional): It allows you to include a conditional statement to filter the items based on a certain condition. Only the items that satisfy the condition will be included in the new list.
Examples
A few examples of using list comprehension in practice, in order from basic to advanced.
Creating a list of squared numbers
This example generates a list of squared numbers from a list of integers:
numbers = [1, 2, 3, 4, 5, 6, 7, 2023]
squared_numbers = [number ** 2 for number in numbers]
print(squared_numbers)
Output:
[1, 4, 9, 16, 25, 36, 49, 4092529]
Filtering a list by a condition
This example creates a list of numbers that are dividable by 3 (greater than 1 and less than 20):
nums = [num for num in range(1, 20) if num % 3 == 0]
print(nums)
Output:
[3, 6, 9, 12, 15, 18]
Creating a complex list
This example uses list comprehension syntax to create a list of tuples containing numbers and their squares:
numbers = [1, 2, 3, 4, 5]
squared_tuples = [(num, num ** 2) for num in numbers]
print(squared_tuples)
Output:
[(1, 1), (2, 4), (3, 9), (4, 16), (5, 25)]
String manipulation
In this example, we’ll build a list of uppercase characters (excluding whitespace) from a given string:
string = "Sling Academy"
uppercase_chars = [char.upper() for char in string if not char.isspace()]
print(uppercase_chars)
Output:
['S', 'L', 'I', 'N', 'G', 'A', 'C', 'A', 'D', 'E', 'M', 'Y']