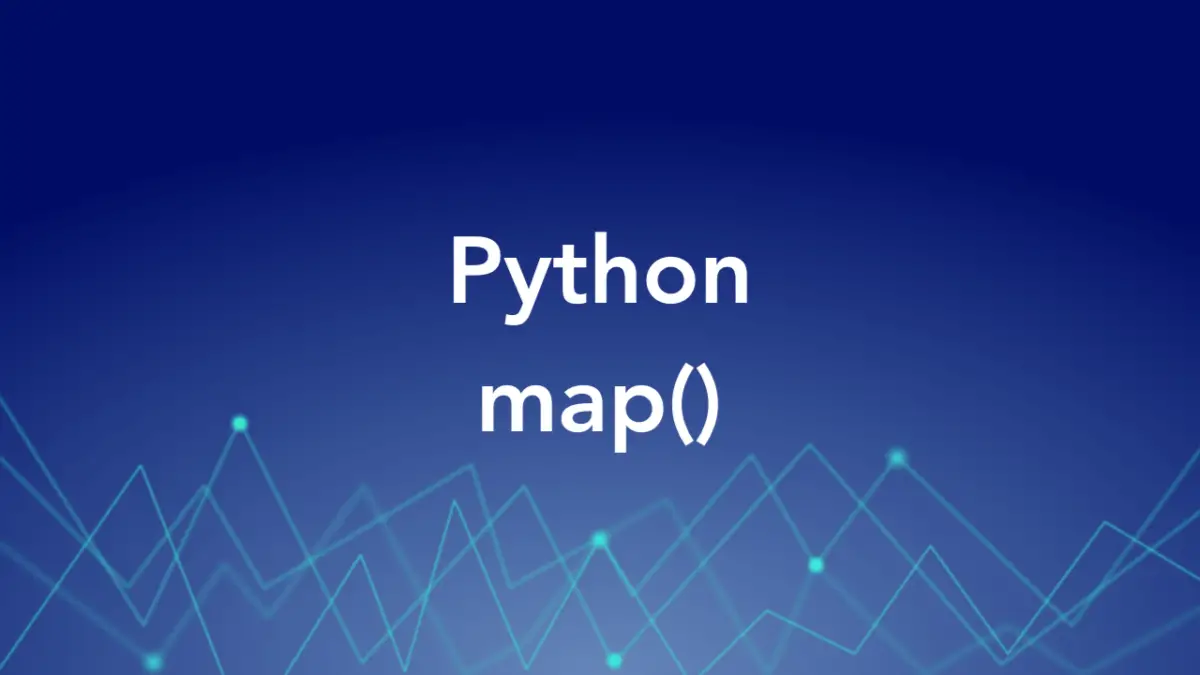
The Fundamentals
map()
is a built-in function in Python that applies a given function to each item in an iterable (e.g., a list, tuple) and returns an iterator that yields the results. Here’s the syntax:
map(function, iterable)
Parameters:
function
: A function that will be applied to each item in the iterable.iterable
: An iterable object, such as a list or tuple, whose elements will be passed to the function.
map()
returns an iterator. To get the results as a list, you can use the list()
function to convert the iterator to a list.
The map()
function provides a concise and efficient way to perform the same operation on multiple elements of a collection without using explicit loops.
Examples
Using a lambda function with map()
In this example, we’ll transform a list of numbers by doubling each element. The point here is to use a lambda function to define the transformation logic and apply it to each element of the list using map()
.
numbers = [1, 2, 3, 4, 5]
transformed_list = list(map(lambda x: x * 2, numbers))
print(transformed_list)
Output:
[2, 4, 6, 8, 10]
Using a named function with map()
In case you have complex and long transformation logic, it’s better to define a separate function rather than using a lambda function. In the following example, we’ll utilize the map()
function to add a unique id
to each element in the list:
import uuid
import pprint
my_list = [
{
"name": "John",
"age": 25,
},
{
"name": "Sally",
"age": 28,
},
{
"name": "Jane",
"age": 32,
},
]
def add_id(e):
e["id"] = uuid.uuid4()
return e
new_list = list(map(add_id, my_list))
pprint.pprint(new_list)
Output:
[{'age': 25,
'id': UUID('593d7ddb-4013-4a3b-a9fb-5672996c9193'),
'name': 'John'},
{'age': 28,
'id': UUID('231c41e4-2e90-4e5d-8c5f-a00a84f90451'),
'name': 'Sally'},
{'age': 32,
'id': UUID('d0fef3ba-54c9-4675-81d8-c607d55b4077'),
'name': 'Jane'}]
That’s it. Happy coding!