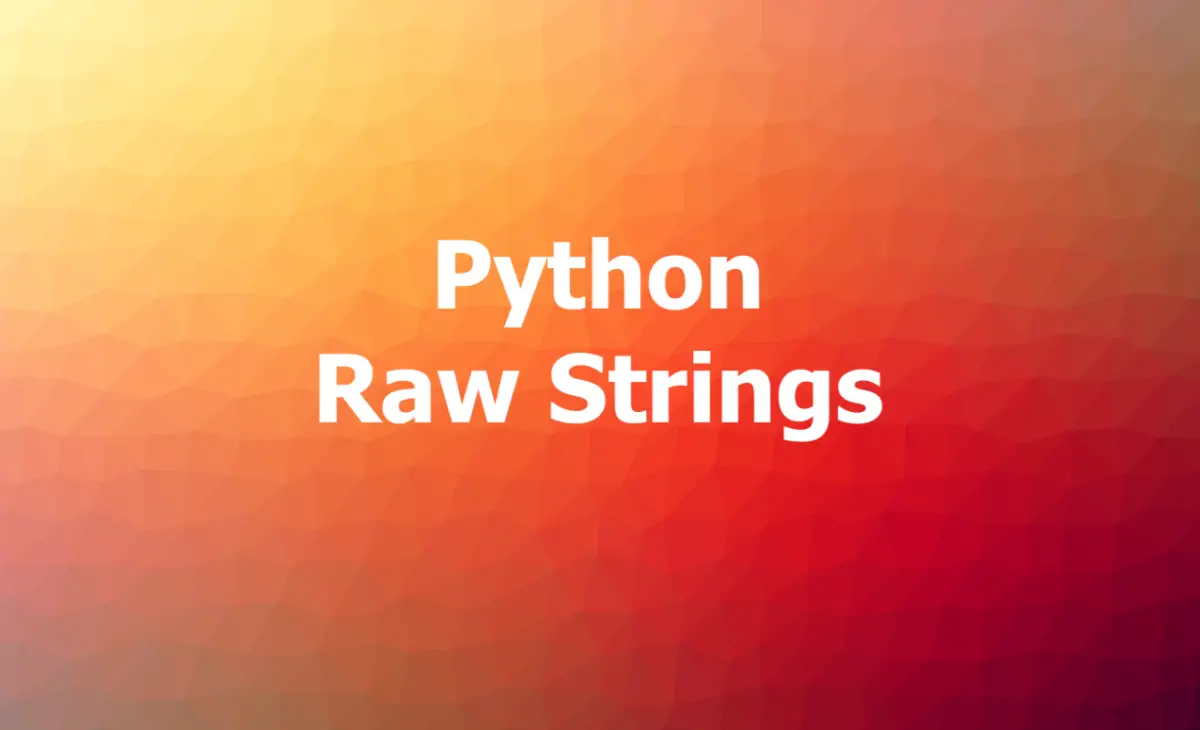
Quick Overview
In Python, raw strings are string literals prefixed with the letter r
. They are used to treat backslashes (/
) as literal characters, ignoring their escape sequence interpretation. Raw strings are commonly used when working with regular expressions, file paths, or any other scenario where backslashes need to be treated as regular characters.
Examples
Some real-world code examples demonstrate the usefulness of raw strings in Python.
File Path
Use a raw string to represent a file path:
file_path = r"C:\Users\Sling Academy\Documents\file.txt"
print(file_path)
Output:
C:\Users\Sling Academy\Documents\file.txt
Raw Strings and Regular Expressions
Using a raw string in a regular expression pattern ensures that backslashes are treated as literal characters, allowing you to write the pattern without additional escaping. This simplifies and increases the readability of your code.
In the example below, the raw string r"\b[A-Z]+\b"
is a regular expression pattern used to match uppercase words in a given text:
import re
# Find all the words that start with a capital letter
pattern = r"\b[A-Z]+\b"
text = "Welcome to SLING ACADEMY. Happy coding!"
matches = re.findall(pattern, text)
print(matches)
Output:
['SLING', 'ACADEMY']
Preserving Double Backslashes as Literal Characters
In the following example, we use a raw string to preserve double backslashes as literal characters:
raw_string = r"This is a raw string with \\ escaped backslashes."
regular_string = "This is a regular string with \\\\ escaped backslashes."
print(raw_string)
print(regular_string)
Output:
This is a raw string with \\ escaped backslashes.
This is a regular string with \\ escaped backslashes.