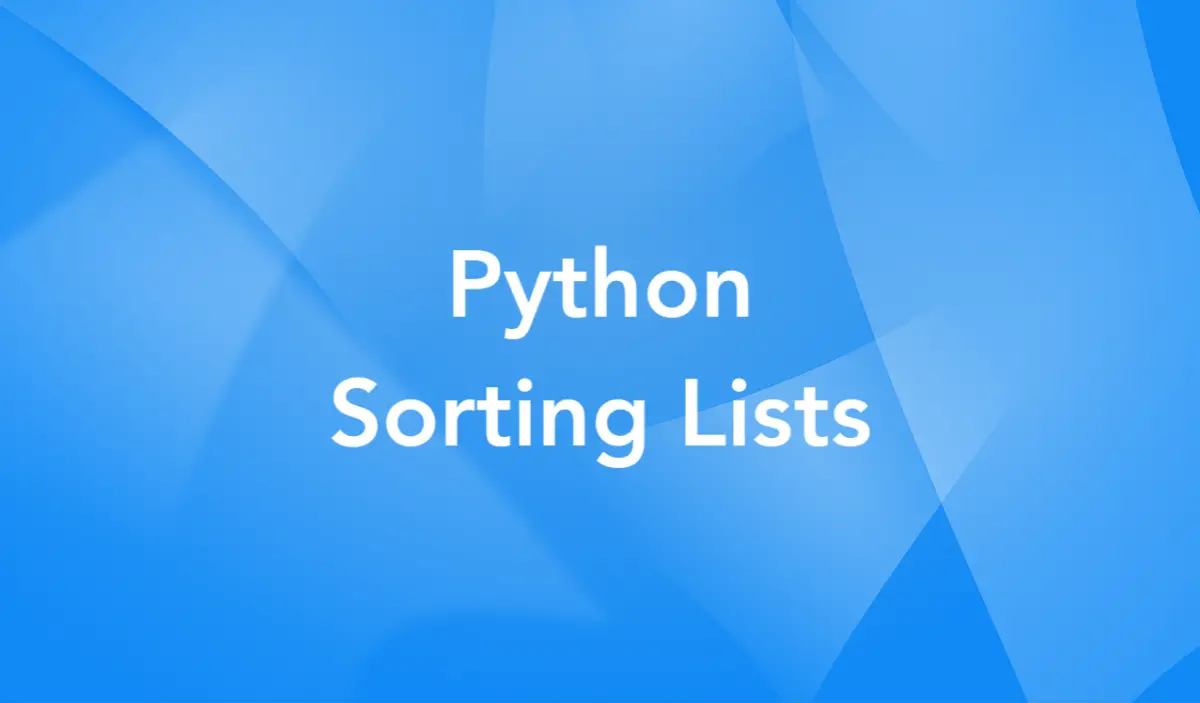
The Fundamentals
The Pythonic way to sort elements in a Python list is to use the sort()
method or the sorted()
function. These two are almost the same, except that the sort()
method modifies the original list in place while the sorted()
function returns a new sorted list (this function can also work with other iterables like tuples and sets).
Syntax & Parameters
Here’s the syntax of the sort()
method:
your_list.sort(key=None, reverse=False)
And here’s the syntax of the sorted()
function:
sorted(your_iterable, key=None, reverse=False)
Let me explain the key
and reverse
parameters (of both):
key
: An optional function that takes one argument and returns a value to be used for sorting. The default value isNone
, which means that the elements are compared directly.reverse
: An optional boolean that indicates whether to sort the list in descending order or not. The default value isFalse
, which means that the list is sorted in ascending order (set it toTrue
if you prefer the descending order).
Performance
Both the sort()
method and the sorted()
function have a time complexity of O(n log n)
, where n
is the number of elements in the input list. This means that they scale quite well with large inputs. The sorting algorithm here is Timsort.
The sort()
method has a space complexity of O(1)
. This tells us that it does not create a new list and only uses a fixed amount of memory. It modifies the list object in place, which can save memory and time if the original list is extremely large.
The sorted()
function has a space complexity of O(n)
. It creates a new list that takes up as much memory as the original object. If you want to keep the original object intact, this function is useful.
Are you getting bored with boring words? It’s time to see some code.
Examples
The following examples are arranged in order from basic to advanced, from simple to complex.
Sorting a List of Numbers (Ascending and Descending)
Here’s the code:
numbers = [3, 2.5, 1, 1.4, 4, 5, 4.1]
# sorting in ascending order
sorted_numbers_asc = sorted(numbers)
print(sorted_numbers_asc)
# sorting in descending order
sorted_numbers_desc = sorted(numbers, reverse=True)
print(sorted_numbers_desc)
Output:
[1, 1.4, 2.5, 3, 4, 4.1, 5]
[5, 4.1, 4, 3, 2.5, 1.4, 1]
Sorting a List of Strings (Case Insensitive)
This example illustrates how to sort a list of words in alphabetical order with case-insensitive:
words = [
'Coconut',
'apple',
'Banana',
'Slingacademy.com',
'TURTLE'
]
# sort the list in alphabetical order (from a to z) ignoring case
words.sort(key=str.lower)
print(words)
Output:
['apple', 'Banana', 'Coconut', 'Slingacademy.com', 'TURTLE']
Sorting a List of Dictionaries
In this example, we’ll sort a list of imaginary products by their price (low to high and high to low):
products = [
{"name": "laptop", "price": 800},
{"name": "phone", "price": 500},
{"name": "tablet", "price": 300},
{"name": "watch", "price": 200},
{"name": "Sling Academy", "price": 0.5}
]
# sort products by price (from low to high)
sorted_products_asc = sorted(products, key=lambda product: product["price"])
print(sorted_products_asc)
# sort products by price (from high to low)
sorted_products_desc = sorted(products, key=lambda product: product["price"], reverse=True)
Output:
# Price low to high
[{'name': 'Sling Academy', 'price': 0.5},
{'name': 'watch', 'price': 200},
{'name': 'tablet', 'price': 300},
{'name': 'phone', 'price': 500},
{'name': 'laptop', 'price': 800}]
# Price high to low
[{'name': 'laptop', 'price': 800},
{'name': 'phone', 'price': 500},
{'name': 'tablet', 'price': 300},
{'name': 'watch', 'price': 200},
{'name': 'Sling Academy', 'price': 0.5}]
Sorting a List of Class Instances
In this example, we will sort a list of class instances (each class object stores information about an employee in a fiction company) by an object attribute:
# define the Employee class
class Employee:
def __init__(self, name, salary):
self.name = name
self.salary = salary
def __repr__(self):
return f"{self.name}: {self.salary}"
# List of employees
employees = [
Employee("Wolf", 5000),
Employee("Turtle", 4000),
Employee("Fire Demon", 6000),
Employee("Kitty", 3000)
]
# Sort the employees by salary in descending order
sorted_employees_desc = sorted(employees, key=lambda e: e.salary, reverse=True)
print(sorted_employees_desc)
Output:
[Fire Demon: 6000, Wolf: 5000, Turtle: 4000, Kitty: 3000]
Conclusion
You’ve learned the fundamentals of the list.sort()
method and the sorted()
function. You’ve also examined a couple of practical examples that demonstrate how to utilize them in real-world use cases. At this point, you’re pretty good to go ahead and solve more complicated tasks related to sorting lists in Python. The tutorial ends here. Good luck!