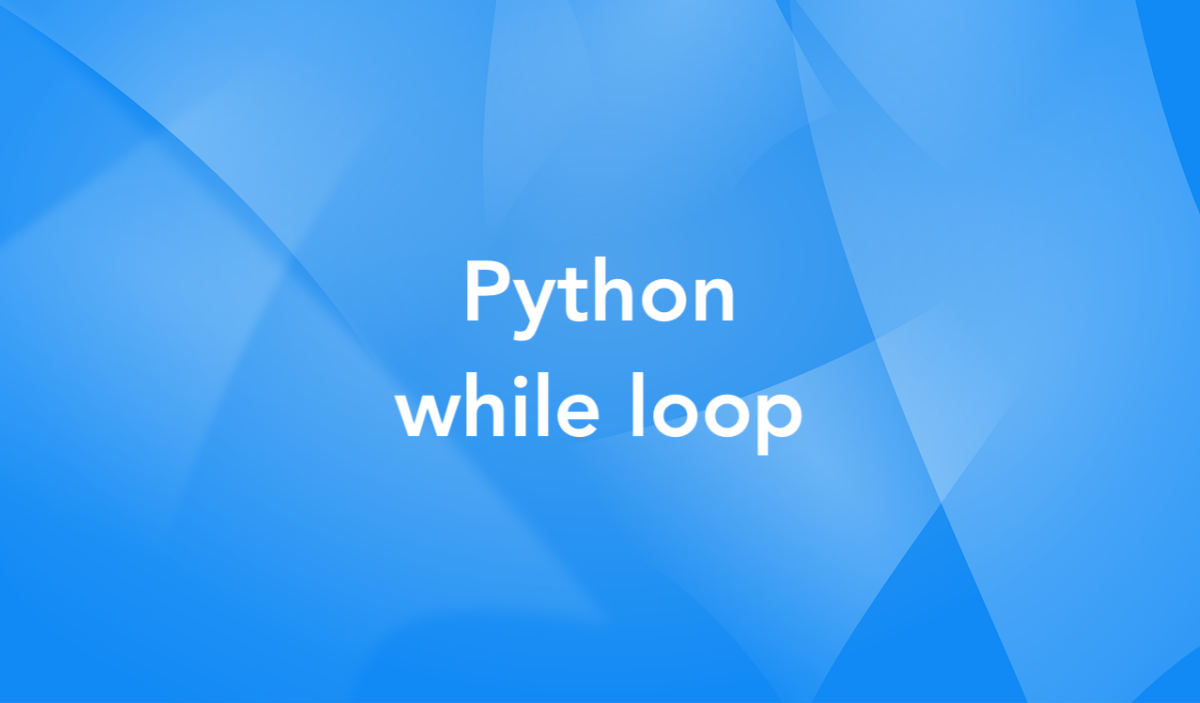
What is the Point?
The while
loop in Python is a type of loop that executes a block of code repeatedly as long as a given condition is True
. Unlike the for loop, which iterates over a fixed sequence of values, the while
loop can run indefinitely until the condition is False
or the loop is interrupted by a break
or return
statement.
General syntax:
while condition:
# code to be executed
# while the condition is true
Explanation:
while
keyword: Used to start awhile
loop.- condition: A Boolean expression that determines whether the loop should continue or exit.
- : colon: Indicates the start of the loop block.
- Indented code block: The block of code that will be executed repeatedly as long as the condition is
True
.
In practice, using the while
loop wisely will make your code much more efficient and concise. Let’s examine some examples for a deeper and better understanding.
Examples
Basic
This example uses a while
loop to print the numbers from 1
to 10
:
# initialize a counter variable
n = 1
# loop until n is greater than 10
while n <= 10:
# print the current value of n
print(n)
# increment n by 1
n = n + 1
Output:
1
2
3
4
5
6
7
8
9
10
In this example, the condition is n <= 10
, which means the loop will run as long as n
is less than or equal to 10
. The body of the loop prints the current value of n
and then increases it by 1
. When n
becomes 11
, the condition becomes False
, and the loop ends.
Infinite Loop with Break
In this example, the while
loop runs indefinitely until the user enters the command quit
. As soon as the input is quit
, the loop will be exited immediately using the break
statement.
while True:
user_input = input("Enter a command: ")
if user_input == "quit":
break
else:
print(f"Executing command: {user_input}")
Output (may vary depending on what you type):
Enter a command: start
Executing command: start
Enter a command: stop
Executing command: stop
Enter a command: quit
While loop with continue statement
This example uses a while
loop to print all the odd numbers from 1
to 20
, skipping the even numbers:
# initialize a counter variable
n = 1
# loop until n is greater than 20
while n <= 20:
# check if n is even
if n % 2 == 0:
# increment n by 1 and skip to the next iteration
n = n + 1
continue
# print the current value of n (odd number)
print(n)
# increment n by 1
n = n + 1
Output:
1
3
5
7
9
11
13
15
17
19
Let me explain the code snippet above. The condition is n <= 20
, which means the loop will run as long as n
is less than or equal to 20
. The body of the loop checks if n
is even using the modulo operator (%
). If n
is even, it increments n
by 1
and uses the continue
statement to skip to the next iteration of the loop without executing the rest of the body. If n
is odd, it prints n
and then increments it by 1
.
While loop with the else clause
This example uses a while
loop with an else
clause to find a prime number between two given numbers:
# define a function to check if a number is prime or not
def is_prime(n):
# check if n is less than or equal to one
if n <= 1:
# return False
return False
# check if n is divisible by any number from two to its square root
for i in range(2, int(n**0.5) + 1):
# if n is divisible by i
if n % i == 0:
# return False
return False
# return True
return True
# define the lower and upper bounds
lower = 10
upper = 20
# loop from lower to upper
while lower <= upper:
# check if lower is prime
if is_prime(lower):
# print lower and break the loop
print("Found a prime number:", lower)
break
# increment lower by one
lower = lower + 1
else:
# print a message if no prime number is found
print("No prime number found between", lower, "and", upper)
Output:
Found a prime number: 11
In this code example, the condition is lower <= upper
, which means the loop will run as long as lower
is less than or equal to upper
. The body of the loop calls the is_prime()
function to check if lower
is a prime number or not. If lower
is prime, it prints it and breaks
the loop. If lower
is not prime, it increments by 1
and continues the loop. The else
clause of the loop executes only if the loop ends normally without a break
statement. In this case, it prints a message that no prime number was found between the given bounds.