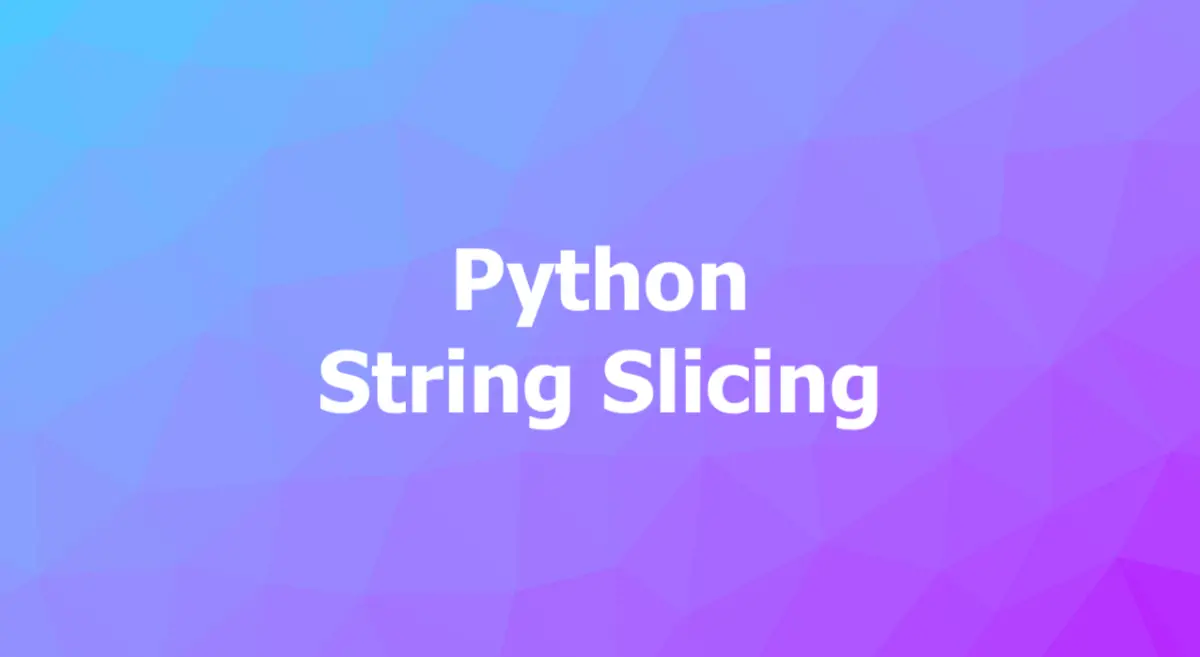
Table of Contents
Overview
In Python, string slicing allows you to extract specific portions, or substrings, from a string by specifying the start and end indices.
Syntax:
string[start:end:step]
Where:
start
is the index indicating where the slice should start (inclusive). If omitted, it defaults to the beginning of the string.end
is the index indicating where the slice should end (exclusive). If not provided, it defaults to the end of the string.step
is an optional parameter that specifies the step or stride value, determining the increment between indices. If omitted, it defaults to 1.
Some main points you should keep in mind when performing string slicing:
- Slicing is a zero-based indexing system, meaning the first character is at index 0.
- Slices are inclusive of the starting index and exclusive of the ending index.
- Negative indices can be used to count from the end of the string (-1 refers to the last character).
The theoretical part is enough here. Let’s look at a few practical examples to get a deeper and better understanding.
Examples
Basic example
text = "Welcome to Sling Academy!"
# Extract a substring starting from index 8 to the end
substring1 = text[8:]
print(substring1) # Output: "to Sling Academy!"
# Extract a substring from index 0 to 7 (exclusive)
substring2 = text[:7]
print(substring2) # Output: "Welcome"
# Extract a substring from index 0 to 5 (exclusive) with a step of 2
substring3 = text[0:5:2]
print(substring3) # Output: "Wlo"
Advanced example
text = "abcdefghijklmnopqrstuvwxyz"
# Reverse the string by using a negative step size
text_reversed = text[::-1]
print(text_reversed) # zyxwvutsrqponmlkjihgfedcba
# Extract every third character from the string
text_third = text[::3]
print(text_third) # adgjmpsvy
# Extract every third character from the reversed string
text_third_reversed = text_reversed[::3]
print(text_third_reversed) # zwtqnkheb
# Extract the middle 10 characters from the string
text_middle = text[8:18]
print(text_middle) # ijklmnopqr
# Extract the middle 10 characters from the reversed string
text_middle_reversed = text_reversed[8:18]
print(text_middle_reversed) # rqpomnlkji