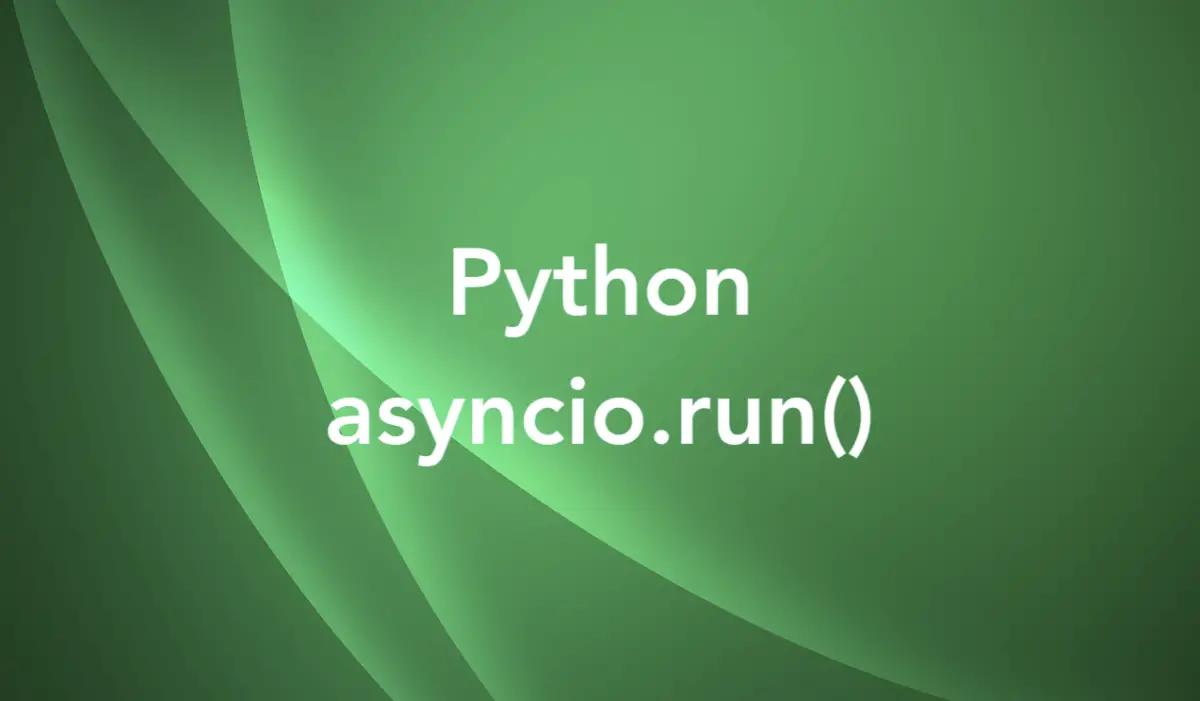
Table of Contents
Overview
The asyncio.run()
function was introduced in Python 3.7 (which was released in 2018). It is a high-level API that creates an event loop, runs the coroutine in the event loop, and finally closes the event loop when the coroutine is complete. It returns the final result of the coroutine or raises an exception if the coroutine fails.
Syntax:
asyncio.run(coro, *, debug=False)
Where:
coro
is a coroutine object that you want to run in an event loop. A coroutine is a special kind of function that can pause and resume its execution using theasync
andawait
keywords.debug
is an optional keyword argument that controls the debug mode of the event loop. IfTrue
, the event loop will log more information, check for common errors, and enable theasyncio.debugger
module. The default value isFalse
.
It is recommended to use the asyncio.run()
function as the main entry point for asyncio
programs unless you need more fine-grained control over the event loop.
Examples
Running a Simple Coroutine
A simple program that prints two parts of a sentence with a delay between:
import asyncio
async def main():
print('Welcome to ...')
await asyncio.sleep(3)
print('... Sling Academy!')
asyncio.run(main())
Asynchronous I/O
This example demonstrates using asyncio.run()
to handle asynchronous I/O operations. It is useful for performing I/O-bound operations concurrently, improving efficiency by not blocking the event loop while waiting for I/O operations to complete.
import asyncio
async def download_file(url):
# Simulate file download
await asyncio.sleep(3)
print(f"Successfully downloaded file from {url}")
async def main():
tasks = [
asyncio.create_task(download_file("https://api.slingacademy.com/v1/sample-data/files/customers.csv")),
asyncio.create_task(download_file("https://api.slingacademy.com/v1/sample-data/files/employees.json")),
]
await asyncio.gather(*tasks)
asyncio.run(main())
In the code above, the download_file()
coroutine uses asyncio.sleep()
to simulate file downloads. In the main()
coroutine, we create tasks for each file download and await their completion using asyncio.gather()
.