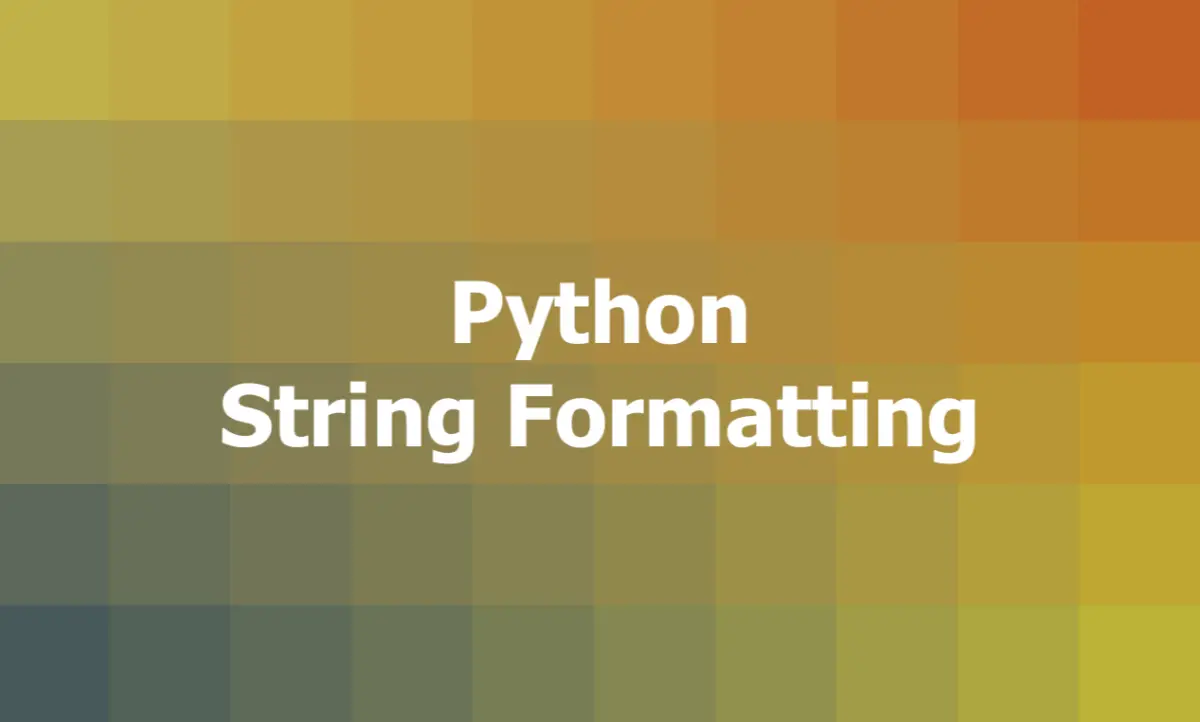
Using f-strings or formatted string literals (Python 3.6+)
This method allows you to embed variables and expressions directly into string literals by prefixing the string with f
(that’s why the term f-strings
was born). Variables and expressions enclosed in curly braces {}
are evaluated, and their values are inserted into the string.
Example:
name = "Robinson Crusoe"
age = 99
message = f"My name is {name} and I am {age} years old. Next year, I will be {age + 1} years old."
print(message)
Output:
My name is Robinson Crusoe and I am 99 years old. Next year, I will be 100 years old.
In general, f-strings are considered the most modern and preferred way of string formatting in Python, as they are concise, expressive, and fast.
Using the str.format() method
This approach allows you to create formatted strings by calling the format()
method on a string and passing values to be inserted. Placeholder curly braces {}
in the string are replaced with the provided values in the order they appear.
Example:
name = "Mr. Turtle"
age = 100
message = "They call me {} and I am {} years old.".format(name, age)
print(message)
Output:
They call me Mr. Turtle and I am 100 years old.
You can also use the str.format(
) method with positional placeholders by specifying the positions of the values to be inserted using curly braces {}
with indices inside:
name = "Wolf"
age = 35
message = "My name is {0} and I am {1} years old.".format(name, age)
print(message)
Output:
My name is Wolf and I am 35 years old.
Besides positional placeholders, you can assign names to the placeholders by using named
arguments in the format()
method. Below is an example of using named placeholders:
name = "Wolf"
age = 35
message = "My name is {name} and I am {age} years old.".format(name=name, age=age)
print(message)
Output:
My name is Wolf and I am 35 years old.
Using the % operator (legacy)
This old-fashioned method uses the %
operator to format strings similar to the C language’s printf-style formatting. The formatting string contains placeholders %s
and %d
that are replaced with the provided values.
Example:
product = "Dog Food"
price = 49.99
description = "This product is is %s and its price is %d bucks." % (product, price)
print(description)
Output:
This product is is Dog Food and its price is 49 bucks.
This method is less and less used in new Python projects because of its cumbersomeness and lack of convenience.
Using the Template class
This is a less common way of string formatting in Python, and it uses the Template
class from the string
module to create template strings that can be substituted with values from a dictionary.
Example:
from string import Template
name = "Wolf"
age = 35
t = Template("Hello, $name. You are $age years old.")
print(t.substitute(name=name, age=age))
Output:
Hello, Wolf. You are 35 years old.