The SciPy library in Python is a core package for scientific computing. It comprises modules for optimization, linear algebra, integration, interpolation, special functions, FFT, signal and image processing, ODE solvers, and more. One of the powerful submodules under SciPy is fft, which is used for fast Fourier transform operations, including the Discrete Sine Transform (DST). In this tutorial, we’ll delve into the dstn()
function within the fft submodule, showcasing its versatility and performance through four illustrative examples.
Working with dstn()
The dstn()
function computes the N-dimensional Discrete Sine Transform. This type of transform is useful in various applications such as signal processing, solving partial differential equations, and image compression. The function can handle multi-dimensional data, making it suitable for complex numerical computations.
Example 1: Basic Usage of dstn()
import scipy.fft
import numpy as np
# Creating a simple array
arr = np.array([1., 2., 3., 4.])
# Applying dstn()
transformed = scipy.fft.dstn(arr)
print(transformed)
This basic example showcases how to apply the dstn()
function to a one-dimensional array. The output would illustrate the transformation of the array into its sine components:
[13.06562965 -5.65685425 5.411961 -4. ]
Example 2: Two-Dimensional Transform
import scipy.fft
import numpy as np
# Create a 2D array
arr2D = np.array([[1, 2], [3, 4]])
# Apply dstn() to a 2D array
transformed_2D = scipy.fft.dstn(arr2D)
print(transformed_2D)
This example extends the application of dstn()
to two-dimensional arrays. You’ll notice how the function elegantly handles data in more complex structures, revealing the multidimensional transformation capabilities.
Output:
[[ 20. -5.65685425]
[-11.3137085 0. ]]
Example 3: Specifying Type and Norm
import scipy.fft
import numpy as np
# Define an array
arr = np.random.rand(4,4)
# Specify DST type-II and normalization
transformed = scipy.fft.dstn(arr, type=2, norm='forward')
print(transformed)
Output (vary):
[[ 2.12288148e-01 -1.64326759e-02 7.90947300e-05 5.46299418e-02]
[-4.42510662e-02 5.40897588e-03 -4.16539123e-02 -1.99223725e-03]
[ 1.17165463e-01 -5.63310297e-02 2.95130194e-02 3.43339279e-03]
[-8.58031839e-03 5.01737454e-03 -3.63401490e-02 1.37737173e-01]]
The Discrete Sine Transform comes in several types, with type-II being the most commonly used. In this example, we specify the type of DST and a normalization mode. Customizing these parameters can be crucial depending on the application context, thus illustrating the function’s flexibility.
Example 4: Applying dstn()
on Real-World Data
This advanced example demonstrates the application of dstn()
on real-world data – such as an image. Due to the complexity and practical importance of this use case, understanding this operation can greatly enhance your proficiency in handling multi-dimensional data sets for signal processing or compression tasks.
from scipy.fft import dstn
import numpy as np
from skimage import data
import matplotlib.pyplot as plt
# Loading a sample image from skimage
image = data.camera()
# Applying DST
transformed_image = dstn(image)
# Displaying the original and transformed image
plt.figure(figsize=(12, 6))
plt.subplot(1, 2, 1)
plt.title('Original')
plt.imshow(image, cmap='gray')
plt.subplot(1, 2, 2)
plt.title('Transformed')
plt.imshow(np.abs(transformed_image), cmap='gray')
plt.show()
Output:
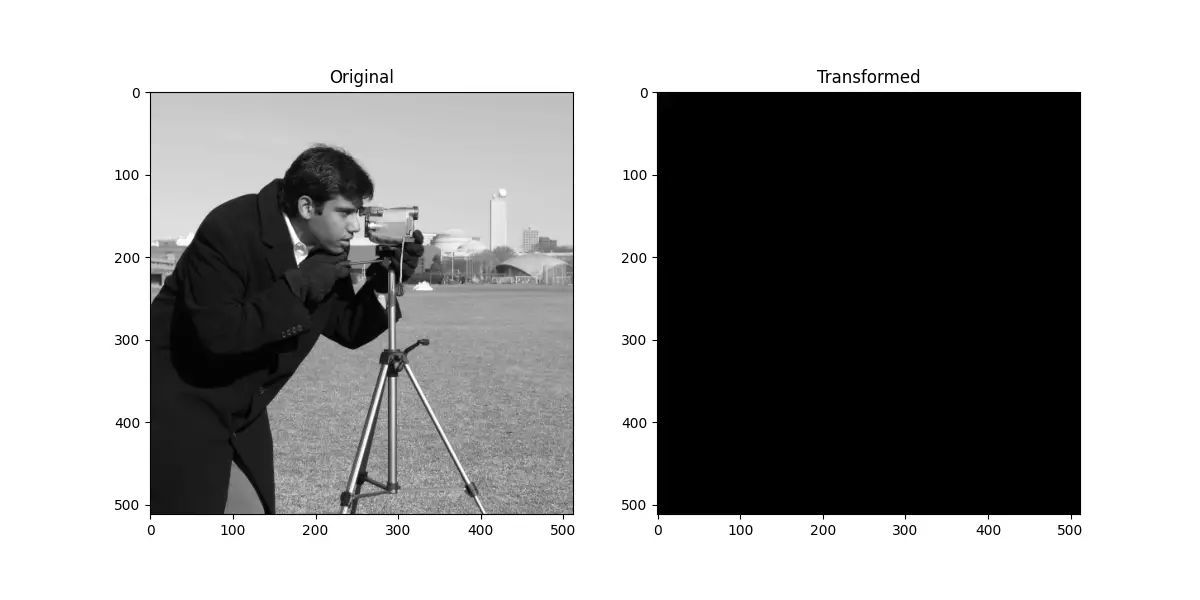
By processing an actual image, this example underscores the capability of dstn()
to work with real-life data, highlighting its practical applications in image analysis and beyond.
Conclusion
In this tutorial, we explored the dstn()
function of SciPy’s fft module through four progressive examples, from simple array transformations to handling complex, multidimensional data. This journey not only showcased the versatility of the dstn()
function but also illuminated its practical applications in real-world scenarios. Whether for academic research, industry tasks, or hobby projects, understanding and utilizing this function can significantly enhance your data processing capabilities.