Table of Contents
Introduction
The SciPy library is an essential toolkit for scientific computing in Python, providing a wide variety of modules for optimization, linear algebra, integration, interpolation, special functions, Fast Fourier Transforms, signal and image processing, Ordinary Differential Equation (ODE) solvers, and more. Among these treasures, the special
module houses various special functions used in mathematical physics and engineering. This tutorial delves into the gammasgn()
function from SciPy’s special
module, elevating your understanding through a series of examples ranging from basic to advanced usage.
What does gammasgn()
Do?
The gammasgn()
function computes the sign of the gamma function without computing the gamma function itself. This can be highly useful in situations where only the sign of the gamma function’s result is needed, potentially saving computational resources. The gamma function extends the factorial function to real and complex numbers. It is defined for all complex numbers except for non-positive integers. Here, we explore how and when to use the gammasgn()
function through varied examples.
Syntax:
scipy.special.gammasgn(x)
Parameters:
- x : array_like – The input value(s) for which the sign of the gamma function is to be computed. It can be a single number or an array of numbers. The input should be real numbers because
gammasgn()
is specifically designed for real inputs.
Returns:
- sign : ndarray or scalar – The sign of the gamma function at each of the input values. The output will be -1, 0, or 1. The function returns 0 for input values where the gamma function is not defined.
Starting with Basics
Example 1: Basic Usage
import scipy.special as sp
x = [0, 0.5, 1, 1.5, 2]
for i in x:
print(f"x = {i}, sign = {sp.gammasgn(i)}")
Output:
x = 0, sign = 0.0
x = 0.5, sign = 1.0
x = 1, sign = 1.0
x = 1.5, sign = 1.0
x = 2, sign = 1.0
In this introductory example, we iterate over a list of real numbers, using the gammasgn()
function to find the sign of the gamma function at each point. This highlights the basic usage and how the function returns 1 for positive values and -1 for negative values of the gamma function. Notably, it returns 1 for integer inputs ≥ 1, coherent with the factorial definition.
Exploring Further
Example 2: Dealing with Negative Inputs
import scipy.special as sp
x = [-3.5, -2.5, -1.5, -0.5]
for i in x:
print(f"x = {i}, sign = {sp.gammasgn(i)}")
Output:
x = -3.5, sign = 1.0
x = -2.5, sign = -1.0
x = -1.5, sign = 1.0
x = -0.5, sign = -1.0
Usage with negative inputs demonstrates the versatility of the gammasgn()
function, where it adeptly handles values that traditional factorial functions could not. This example aims to showcase handling negative arguments, emphasizing the function’s capability to calculate sign for a broader range of values.
Advanced Applications
Example 3: Visualization of gammasgn()
Over a Range
import numpy as np
import scipy.special as sp
import matplotlib.pyplot as plt
x = np.linspace(-5, 5, 400)
y = sp.gammasgn(x)
plt.plot(x, y)
plt.title('Sign of the Gamma Function Over a Range')
plt.xlabel('x')
plt.ylabel('Sign of the Gamma Function')
plt.grid(True)
plt.show()
Output:
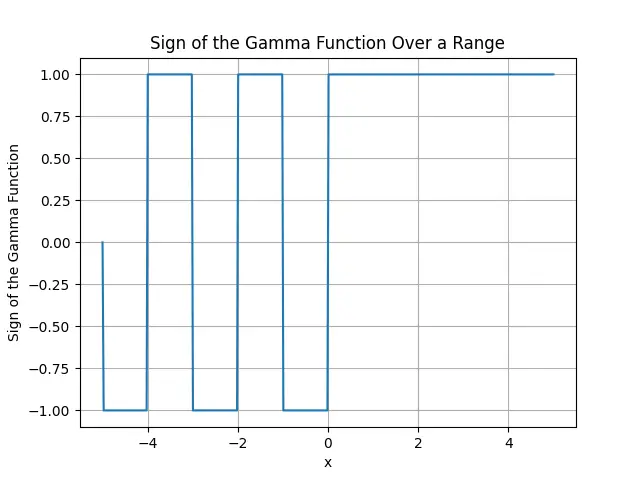
This example steps beyond simple calculations, utilizing gammasgn()
to generate data for visualization. Using numpy.linspace()
to create a range of values and matplotlib to plot, this demonstrates an advanced application of the function, visualizing the sign changes of the gamma function across a continuous range of values.
Example 4: Conditional Evaluation with Gamma Function Sign Influence
Let’s consider a hypothetical scenario where you need to evaluate a function that behaves differently depending on the sign of the gamma function at different points. This function could be part of a larger computation, such as in statistical physics or other fields where the gamma function arises naturally.
For this example, assume we have a function f(x)
that incorporates the gamma function in its calculation. The behavior of f(x)
will depend on the sign of the gamma function at x
. Specifically:
- If the sign of the gamma function at
x
is positive,f(x)
will include an additional factor based onx
. - If the sign is negative,
f(x)
will involve a different factor.
import numpy as np
from scipy.special import gammasgn, gamma
import matplotlib.pyplot as plt
# Define our complex function f(x)
def f(x):
gamma_sign = gammasgn(x)
if gamma_sign > 0:
# When the gamma function's sign is positive
return gamma(x) * np.cos(x)
else:
# When the gamma function's sign is negative
return gamma(x) * np.sin(x)
# Evaluate this function over a range of x values
x_values = np.linspace(-5, 5, 400)
y_values = np.array([f(x) for x in x_values])
# Plot the real part of the function
plt.figure(figsize=(10, 6))
plt.plot(x_values, y_values.real, label='Real part of f(x)')
plt.title('Function Evaluation Based on the Sign of the Gamma Function')
plt.xlabel('x')
plt.ylabel('Real part of f(x)')
plt.legend()
plt.grid(True)
plt.show()
# Plot the imaginary part of the function
plt.figure(figsize=(10, 6))
plt.plot(x_values, y_values.imag, label='Imaginary part of f(x)', color='orange')
plt.title('Function Evaluation Based on the Sign of the Gamma Function')
plt.xlabel('x')
plt.ylabel('Imaginary part of f(x)')
plt.legend()
plt.grid(True)
plt.show()
Output (you’ll see 2 graphs):
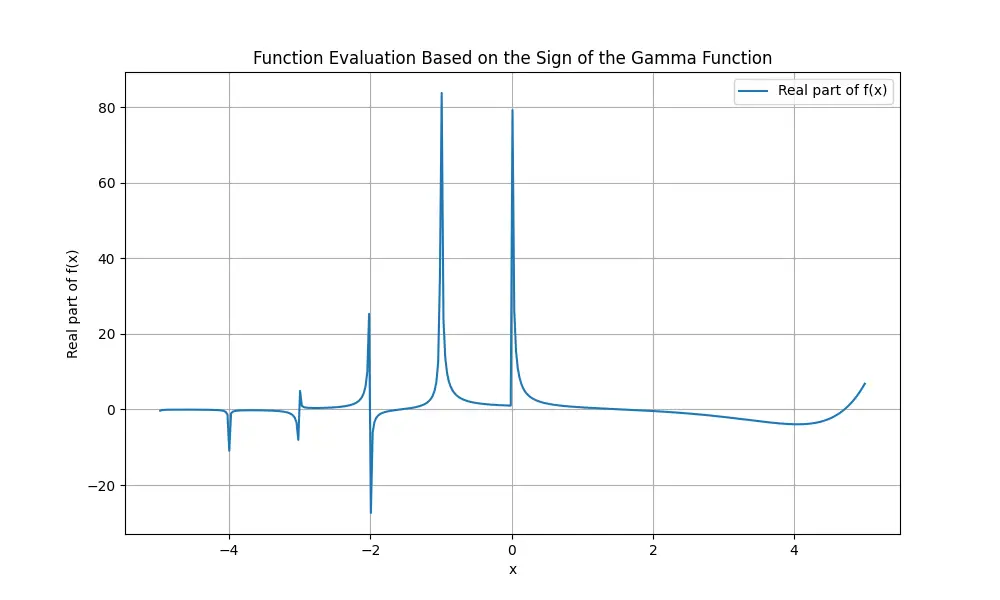
And:
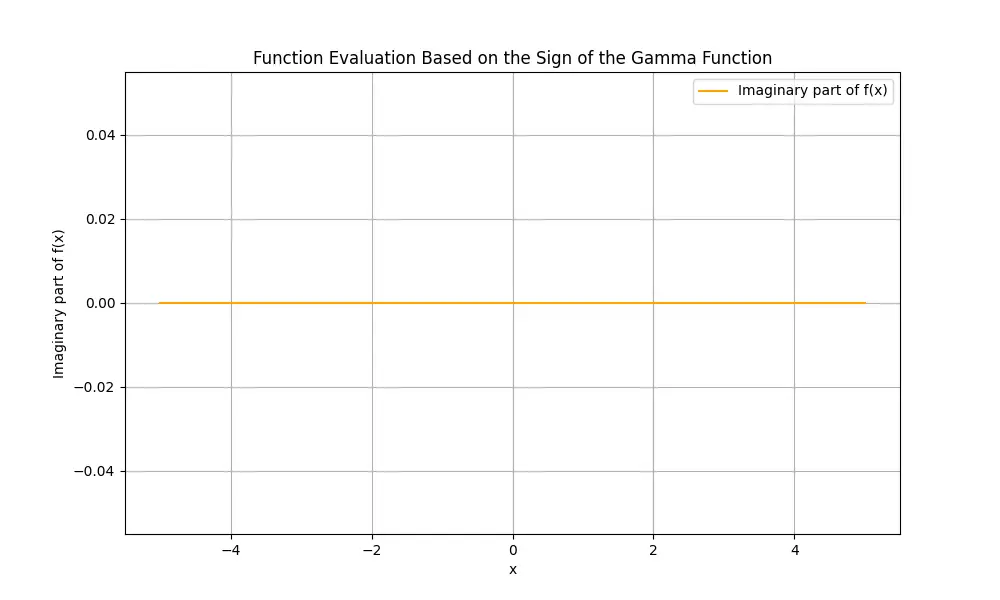
This example is purely illustrative to demonstrate how gammasgn()
might be incorporated into a larger, more complex calculation. The actual mathematical or physical significance of the function f(x)
as defined here is hypothetical and serves to showcase how the sign of the gamma function can influence computational paths within a function. In practice, the use of gammasgn()
and the gamma function would be dictated by the specific requirements of the problem you’re addressing.
Conclusion
Through a series of progressively complex examples, we’ve explored the utility and versatility of the gammasgn()
function within SciPy’s special
module. From basic numerical inputs to advanced visualization and handling of complex numbers, gammasgn()
proves to be an essential function for computational mathematics. Whether for academic research or industrial applications, understanding and utilizing gammasgn()
can significantly contribute to an efficient and deeper understanding of mathematical computations involving the gamma function.